7.6.2. HTTP Messaging¶
Table of contents
Provides a function to send and receive messages using HTTP.
The data model shown in Data model of sent and received messages is assumed for HTTP messaging. Also, General Data Format is used for the message format.
Important
In the Data model of sent and received messages, the framework control header is an item specified independently by Nablarch, and it is assumed to be included in the message body.
There is no problem if the message format can be designed in the project, but the requirement may not be met if the message format is already specified by the external system.
Therefore, using the following functions is recommended.
- The use of RESTful Web service is recommended for the server (message receive).
- The use of Client function provided by JSR339 (JAX-RS2.0) is recommended for client (message send).
If the use of this function is unavoidable, refer to Change the reading and writing of the framework control header and handle by adding the implementation in the project.
The assumed runtime platform differs for HTTP messaging depending on the type of send and receive.
Type of send and receive | Runtime platform |
---|---|
HTTP receive message | HTTP messaging |
HTTP send message | Does not depend on the runtime platform |
7.6.2.1. Function overview¶
7.6.2.1.1. Can be prepared in the same way as MOM Messaging¶
Message send/receive is implemented in HTTP messaging using the same API as MOM Messaging given below. Therefore, if users have experience in using MOM Messaging, implementation time can be minimized.
7.6.2.2. Module list¶
<dependency>
<groupId>com.nablarch.framework</groupId>
<artifactId>nablarch-fw-messaging</artifactId>
</dependency>
<dependency>
<groupId>com.nablarch.framework</groupId>
<artifactId>nablarch-fw-messaging-http</artifactId>
</dependency>
7.6.2.3. How to use¶
7.6.2.3.1. Configure settings to use HTTP messaging¶
In the case of receive message, no special configuration is required other than the handler configuration of the runtime platform.
In the case of send message, add the following classes to the component definition.
- MessageSenderClient implementation class (HTTP send/receive)
A configuration example is shown below.
- Point
- HttpMessagingClient is provided as the default implementation of MessageSenderClient.
- Component name is specified as
messageSenderClient
because it is used as a lookup.
<component name="messageSenderClient"
class="nablarch.fw.messaging.realtime.http.client.HttpMessagingClient" />
7.6.2.3.2. Receive message (HTTP receive message)¶
Receive a message from an external system and send a response.
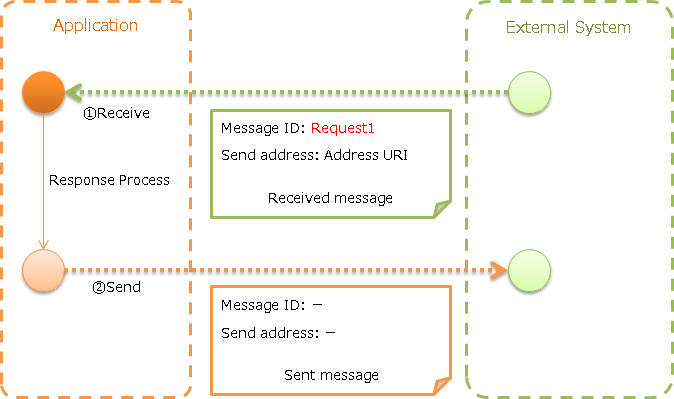
- Implementation examples
- Point
- HTTP message receive is created with MessagingAction.
- Create the response message with RequestMessage.reply.
public class SampleAction extends MessagingAction { protected ResponseMessage onReceive(RequestMessage request, ExecutionContext context) { // Receive data process Map<String, Object> reqData = request.getParamMap(); // (Omitted) // Returns response data return request.reply() .setStatusCodeHeader("200") .addRecord(new HashMap() {{ // Content of message body put("FIcode", "9999"); put("FIname", "Nablarch bank"); put("officeCode", "111"); /* * (Rest is omitted) */ }}); } }
7.6.2.3.3. Send message (HTTP send message)¶
Send a message to an external system and receive the response. Wait until a response message is received or the wait timeout expires.
If a timeout occurs because a response cannot be received within the specified time, a compensation process needs to be performed.
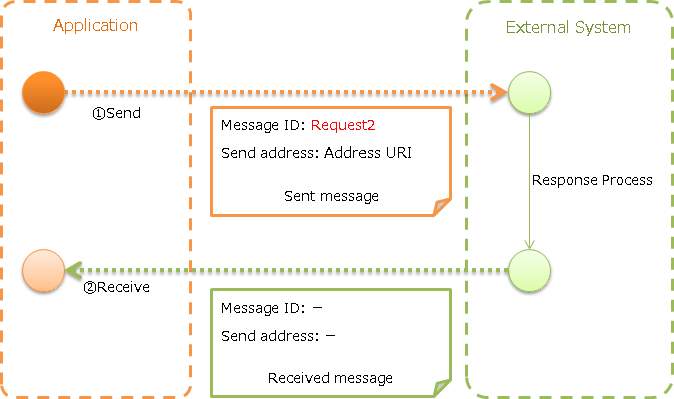
- Implementation examples
- Point
- Create the request message with SyncMessage.
- To send a message, use MessageSender#sendSync. For details of how to use, refer to the linked Javadoc.
// Create a request message SyncMessage requestMessage = new SyncMessage("RM11AC0202") // Configure the message ID .addDataRecord(new HashMap() {{ // Content of message body put("FIcode", "9999"); put("FIname", "Nablarch bank"); put("officeCode", "111"); /* * (Rest is omitted) */ }}) // Send request message SyncMessage responseMessage = MessageSender.sendSync(requestMessage);
To send an unique text as HTTP header, configure in the header record of the message created as follows.
// Message header content requestMessage.getHeaderRecord().put("Accept-Charset", "UTF-8");
7.6.2.4. Expansion example¶
7.6.2.4.1. Change the reading and writing of the framework control header¶
In some cases, reading and writing of the framework control header may require to be changed when the message format is already defined in the external system. To support this, add the implementation in the project. The following shows the support method for each type of send and receive.
- For HTTP send message
- The framework control header is read and written according to the format definition of the message body. Therefore, the format definition of the message body may be changed according to the content.
- For HTTP receive message
Reading and writing to the framework control header is performed by the class with the FwHeaderDefinition interface implementation. StandardFwHeaderDefinition is used by default.
Therefore, referring to StandardFwHeaderDefinition, a class that implements the FwHeaderDefinition interface is created in the project and configured in HTTP Messaging Request Conversion Handler and HTTP Messaging Response Conversion Handler.
Tip
Whether to use the framework control header is optional. Framework control header need not be used unless there are special requirements.
7.6.2.4.2. Change the HTTP client process of HTTP send message¶
As explained in Configure settings to use HTTP messaging, HttpMessagingClient is used for HTTP send message.
HttpMessagingClient
performs various processes as an HTTP client.
For example, Accept: text/json,text/xml
is fixedly configured in the HTTP header of the message to be sent.
If the default operation of HttpMessagingClient does not meet the project requirements, customize by creating a class that inherits HttpMessagingClient and configuring to the component definition with the method given in Configure settings to use HTTP messaging.
7.6.2.5. Data model of sent and received messages¶
In HTTP messaging, the contents of sent and received messages are expressed with the following data model.
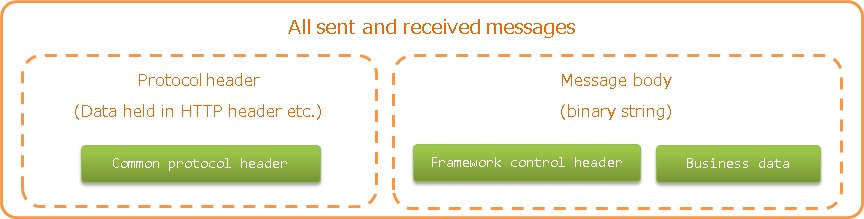
- Protocol header
- This header area mainly stores information used in message send and receive process of web container. The protocol header can be accessed with the Map interface.
- Common protocol header
The following headers among the protocol headers used by the framework can be accessed with a specific key name. The key name is shown in parentheses.
- Message ID (X-Message-Id)
Uniquely numbered string for each message
Sending: Value numbered during send process Receiving: Value issued by the sender - Correlation message ID (X-Correlation-Id)
Message ID of the message to which the message is related
Response message: Message ID of request message Resend request: Message ID of request message requesting the resend of response
- Message body
The data area of the HTTP request is called the message body. The framework function uses only the protocol header area in principle. The other data areas are handled as unanalyzed simple binary data.
The message body is analyzed by General Data Format. This enables reading and writing the content of the message in Map format with the field name as a key.
- Framework control header
Many of the functions provided by this framework are designed on the assumption that specific control items are defined in the message. Such control items are called
framework control headers
.The correspondence between the framework control header and the handler using it are as follows.
- Request ID
ID to identify the business process that should be executed by the application that received this message.
Main handlers that use this header:
- User ID
A character string that indicates the execution permission of this message
Main handlers that use this header:
- Resend request flag
Flag set when sending a resend request message
Main handlers that use this header:
- Status code
Code value that represents the processing result for the request message
Main handlers that use this header:
The framework control header must be defined with the following field names in the first data record of the message body by default.
Request ID: requestId User ID: userId Resend request flag: resendFlag Status code: statusCode The following is an example of a standard framework control header definition.
#=================================================================== # Framework control header part (50 bytes) #=================================================================== [NablarchHeader] 1 requestId X(10) # Request ID 11 userId X(10) # User ID 21 resendFlag X(1) "0" # Resend request flag (0: Initial send 1: Resend request) 22 statusCode X(4) "200" # Status code 26 ?filler X(25) # Reserve area #====================================================================
When items other than the framework control header are included in the format definition, the items can be accessed as optional header items of framework control header and used for the purpose of simple expansion of the framework control header for each project.
It is highly recommended to provide a reserve area to add headers that are required to manage optional items and framework functions that may be added in the future.