6.4.2. JAX-RS BeanValidation Handler¶
Table of contents
This handler executes Bean Validation for Form (Bean) received by the resource (action) class. If a validation error occurs during validation, the process is not delegated to the subsequent handler, and the process is ended after sending ApplicationException.
This handler performs the following process.
- Performs Bean Validation for the form received by the resource (action) class method.
The process flow is as follows.
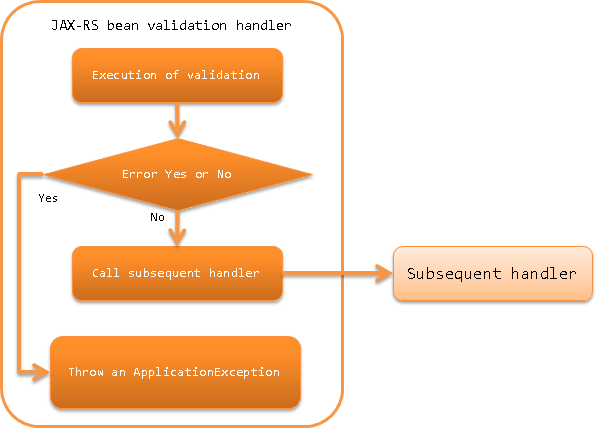
6.4.2.2. Module list¶
<dependency>
<groupId>com.nablarch.framework</groupId>
<artifactId>nablarch-fw-jaxrs</artifactId>
</dependency>
<!-- Bean Validation module -->
<dependency>
<groupId>com.nablarch.framework</groupId>
<artifactId>nablarch-core-validation-ee</artifactId>
</dependency>
6.4.2.3. Constraints¶
- Configure this handler after the Request Body Conversion Handler
- Since this handler is for the validation of Form (Bean) converted from the request body by the Request Body Conversion Handler.
6.4.2.4. Execute validation for Form (Bean) received by resource (action)¶
To validate the Form (Bean) received by the resource (action) method, configure Valid annotation for that method.
An example is shown below.
// Since validation has to be performed on the Person object,
// configure a Valid annotation.
@POST
@Consumes(MediaType.APPLICATION_JSON)
@Valid
public HttpResponse save(Person person) {
UniversalDao.insert(person);
return new HttpResponse();
}
6.4.2.5. Specify Group of Bean Validation¶
A Bean Validation group can be specified by setting the ConvertGroup annotation for the method with the Valid annotation set.
The from
and to
attributes must be specified for the ConvertGroup annotation.
Each must be specified as follows.
from
: Specify Default.class as a fixed value.to
: Specify the group of Bean Validation.
An example is shown below.
// Among the validation rules set within the Person class,
// only the rules belonging to the Create group are used for validation.
@POST
@Consumes(MediaType.APPLICATION_JSON)
@Valid
@ConvertGroup(from = Default.class, to = Create.class)
public HttpResponse save(Person person) {
UniversalDao.insert(person);
return new HttpResponse();
}