CORS Preflight Request Handler¶
Table of contents
This handler is used to implement Cross-Origin Resource Sharing (CORS) with RESTful web service.
Implementing CORS requires handling of the preflight request which is sent before the actual request and the actual request. Preflight requests are handled by this handler, and handling for the actual request is handled by CorsResponseFinisher, which implements ResponseFinisher as described in Add common processing to the response returned to the client.
This handler performs the following process.
- Return the response to the preflight request if the request is a preflight request.
The process flow is as follows.
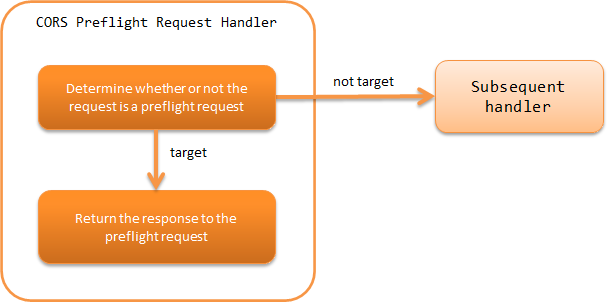
Module list¶
<dependency>
<groupId>com.nablarch.framework</groupId>
<artifactId>nablarch-fw-jaxrs</artifactId>
</dependency>
Constraints¶
- Configure this handler after the Jakarta RESTful Web Services Response Handler
- The HttpResponse created by this handler is handled by Jakarta RESTful Web Services Response Handler, so the this handler should be placed after Jakarta RESTful Web Services Response Handler.
Implementing CORS¶
To implement CORS, configure this handler and CorsResponseFinisher.
CORS is handled by the Cors interface. The framework provides the BasicCors class as a basic implementation of CORS. Just specify BasicCors for this handler and CorsResponseFinisher.
The settings are shown below.
<!-- BasicCors -->
<component name="cors" class="nablarch.fw.jaxrs.cors.BasicCors">
<!-- Specifies which Origin to allow. This setting is required -->
<property name="allowOrigins">
<list>
<value>https://www.example.com</value>
</list>
</property>
</component>
<!-- Handler queue configuration -->
<component name="webFrontController" class="nablarch.fw.web.servlet.WebFrontController">
<property name="handlerQueue">
<list>
<!-- Other handlers are omitted -->
<!-- JaxRsResponseHandler -->
<component class="nablarch.fw.jaxrs.JaxRsResponseHandler">
<property name="responseFinishers">
<list>
<!-- CorsResponseFinisher -->
<component class="nablarch.fw.jaxrs.cors.CorsResponseFinisher">
<!-- Specifies BasicCors -->
<property name="cors" ref="cors" />
</component>
</list>
</property>
</component>
<!-- CorsPreflightRequestHandler -->
<component class="nablarch.fw.jaxrs.CorsPreflightRequestHandler">
<!-- Specifies BasicCors -->
<property name="cors" ref="cors" />
</component>
</list>
</property>
</component>
BasicCors performs the following processing by default.
- Preflight Request (the process called by CorsPreflightRequestHandler)
A request is considered a preflight request if it satisfies all of the following conditions
- HTTP method:OPTIONS
- Origin header:exist
- Access-Control-Request-Method header:exist
Return the following response if the request is a preflight request
- Status code:204
- Access-Control-Allow-Methods header:OPTIONS, GET, POST, PUT, DELETE, PATCH
- Access-Control-Allow-Headers header:Content-Type, X-CSRF-TOKEN
- Access-Control-Max-Age header:-1
- The same response header as in the ” Actual Request” below is also set.
- Actual Request (the process called by CorusResponseFinisher)
Set the following response headers
Access-Control-Allow-Origin header:Origin header of the request
- Set this header only if the request’s Origin header is included in the allowed Origin
Vary header:Origin
- Set this header only if the request’s Origin header is included in the allowed Origin
Access-Control-Allow-Credentials header:true
Among the default processes, the contents of the response header can be changed in the settings. See Javadoc in BasicCors for what can be changed in the configuration.